Project Type: Solo
Technology Stack: C++, SDL
Role: Programmer
GitHub: SDL Engine
Demo
Description
After creating an asteroids game using SFML and not following any architecture patterns, I decided to write my custom game engine. The project is done completely from scratch using only SDL and C++. The main objective was to showcase all the skills I currently possess in areas such as Software Architecture and C++ programming.
I came up with the idea of replicating the “Neo Bomberman” game from 1997, while also adding other competitive elements to the game such as collecting coins for a limited time. As the result of such a mix, the main target is to get as many points as possible while keeping the hero alive. But there is a still possibility to risk and play the old-fashioned way by reflecting bombs and trying to kill the opponent.
The most complex features implemented include:
- Scene management
- Object management + Component system
- Animation system
- Physics collision and collision response (different types of objects such as kinematic, colliders and triggers)
- Input manager for 2 players using callback functions (std::bind and std::function)
- JSON Serialization of everything (including scenes, objects inside of it and even components)
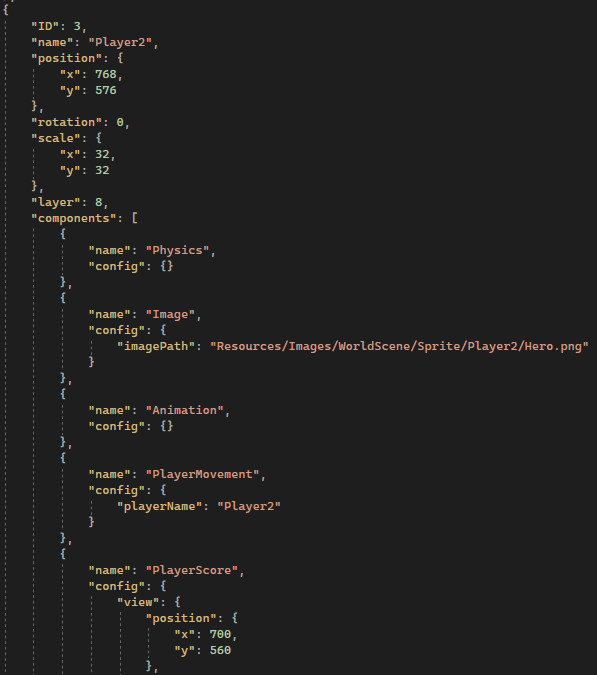
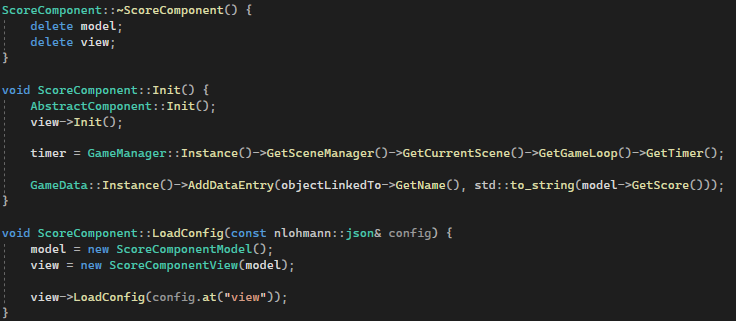
To showcase knowledge of optimization techniques, I used:
- Singletons (Logging)
- Service Locators (Audio)
- Factory method (GameObjects and Components creation)
- Object Pool
- Separate update from a render in the game loop
- Reusing assets (e.g. reusing the same animation texture for multiple objects)
- Optimized container choice (unordered_maps and vectors)
To Improve
Overall, even though I find this project highlights my current skills and shows my understanding of different aspects of game programming, there are multiple things to improve. They are:
- Replace raw pointers with smart pointers
- Improve the split between the engine and the game mechanics by creating an additional factory for custom components (or work with the engine as a separate module).
- Improve physics (layer mask and broad phase using node check, utilize SAT)
- Lacking documentation